- Published on
Kubernetes Development & CI/CD with Skaffold
- Authors
- Name
- Parminder Singh
Building for Kubernetes is complex. For any fairly complex app that has multiple services, developing, building and deploying these services in a standard fashion can be a challenge. Skaffold is a tool that simplifies this process by automating the build, push, and deploy process, making it easier for teams to develop and deploy applications consistently. In this article, I will discuss how Skaffold solves common problems in developing in the Kubernetes environment and standardizes development across teams with a simple example, even though there's nothing simple about Kubernetes :D.
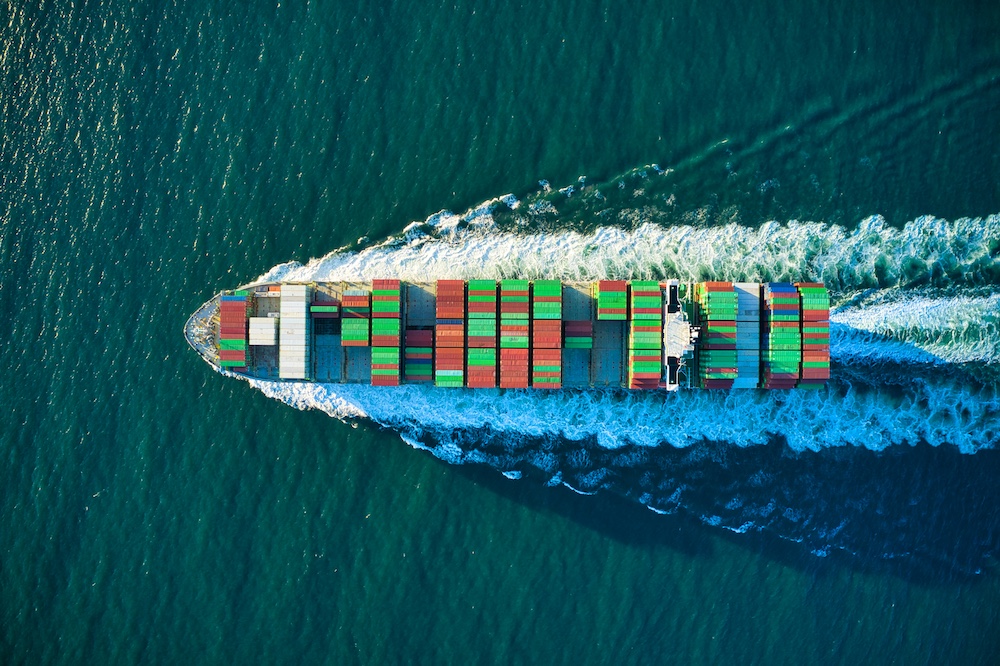
Photo by Venti Views on Unsplash
Let's start with some common challenges developers face when working with Kubernetes:
- Slow Development Cycle: Every code change requires rebuilding images, pushing them to a registry, and redeploying.
- Environment Inconsistencies: Working with different environments (local, staging, production, etc), can lead to discrepancies in how applications behave if they are not configured correctly.
- Difficult Debugging: Debugging applications inside Kubernetes clusters is complex and time-consuming.
- Complicated CI/CD Pipelines: Writing and maintaining custom scripts for building, tagging, and deploying container images is tedious and error-prone.
Skaffold
Skaffold is an open-source tool by Google that simplifies Kubernetes application development. It automates the build, push, and deploy process, making it easier for teams to develop and deploy applications consistently.
Features I will cover in this article
- Continuous Development Mode (
skaffold dev
): Automatically builds and deploys apps when changes are made. - Profiles: Enables different configurations for development, testing, and production environments.
- Remote Debugging: Simplifies debugging applications running in a Kubernetes cluster.
- CI/CD Integration: Automates container builds and Kubernetes deployments in pipelines like GitLab CI/CD.
To demonstrate the idea, I created a GitHub project that you can checkout here. The project contains a simple Python backend and a React frontend, both running in Kubernetes. The backend serves a simple API, and the frontend displays the API response. The main branch is simple and doesn't use Skaffold, while the skaffold branch uses Skaffold to automate the development and deployment process. README file lists all the commands you need to run to get the project up and running. I used minikube on my mac, but you can use any Kubernetes cluster you have access to.
skaffold dev
1. Continuous Development with In a standard Kubernetes workflow, when you modify your code, you need to:
- Rebuild the container image (
docker build
) - Push the image to a container registry (
docker push
) - Update the Kubernetes manifests with the new image tag
- Deploy the changes (
kubectl apply -f
)
With skaffold dev
, Skaffold automates this process by:
- Watching for file changes
- Rebuilding and pushing only the modified parts
- Syncing changes to running containers without full restarts
- Automatically applying Kubernetes updates
Running Skaffold Dev Mode
Below is the skaffold.yaml
file that defines the build and deploy configuration for the backend and frontend services
apiVersion: skaffold/v2beta28
kind: Config
build:
artifacts:
- image: backend-image
context: backend
- image: frontend-image
context: frontend
deploy:
kubectl:
manifests:
- k8s/*.yaml
portForward:
- resourceType: service
resourceName: frontend-service
port: 80
localPort: 8888
Run Skaffold in development mode:
skaffold dev
Check this video out. I made a change in the backend code, and Skaffold automatically rebuilds the image, pushes it to the registry, and updates the deployment in Kubernetes. I pretty much just saved the file, and Skaffold did the rest.
2. Remote Debugging
There's no straightforward way to debug applications running in Kubernetes. Logs can be distributed across multiple pods.
Skaffold provides port-forwarding and debugging hooks, allowing developers to interact with services inside Kubernetes as if they were running locally. Skaffold as of Mar 2025 supports debugging with Go, Java, Node.js, and Python.
Simply run skaffold debug
to start a debugging session:
skaffold dev
Check this video where I set a breakpoint the node code and debug it using Skaffold.
3. Using Profiles for Different Environments
With Skaffold's profiles we can define different configurations for each environment within the same skaffold.yaml file. The underlying Kubernetes manifests remain separate, but skaffold profiles streamline the process of selecting and deploying the right configuration for a given environment.
profiles:
- name: dev
build:
local: {}
deploy:
kubectl:
manifests:
- k8s/dev/*.yaml
- name: prod
build:
googleCloudBuild: {}
deploy:
kubectl:
manifests:
- k8s/prod/*.yaml
Now, to deploy to dev, run:
skaffold run -p dev
For production, run:
skaffold run -p prod
4. CI/CD with GitLab Using Skaffold
Skaffold simplifies Kubernetes deployments in CI/CD environments by handling builds, tagging, and deployments. It provides "building-blocks" to facilitate a structured CI/CD pipeline.
skaffold run: A single command that handles building, tagging, pushing, deploying, and monitoring deployments, ideal for simple CD setups.
skaffold build: Builds and tags container images, optionally pushing them to a registry.
skaffold deploy: Deploys pre-built images to a Kubernetes cluster using manifests, Helm, or Kustomize.
skaffold render: Outputs Kubernetes manifests with resolved image references, useful for GitOps workflows.
skaffold apply: Applies pre-rendered manifests to a Kubernetes cluster.
skaffold status-check: Ensures that deployments stabilize before marking the pipeline successful.
Read more here.
.gitlab-ci.yml
Example: GitLab CI/CD Pipeline with Skaffold stages:
- build
- deploy
docker-build:
stage: build
script:
- skaffold build
deploy:
stage: deploy
script:
- skaffold deploy
environment:
name: production
url: http://singh-app.com
Skaffold removes friction in Kubernetes development and deployment by automating repetitive tasks, standardizing workflows, and supporting multiple environments. Have you used Skaffold? Or another alternative? I'd love to hear your thoughts.