- Published on
Are you building AI Agents
- Authors
- Name
- Parminder Singh
Oracle recently announced that 50+ role-based AI agents within the Oracle Fusion Cloud Applications Suite will help successfully execute frequent, repetitive tasks. Other companies are doing the same [1, 2, 3]. In this article I will discuss what AI agents are, what are some of the use cases and link some tools/frameworks that can help you design and build agents.
An AI agent is a goal oriented autonomous software capable of perception, decision making and action. It can gather information about its parameters and environment through sensors or data feeds, process this information to choose the best course of action based on its goals and execute the chosen action (or actions). It is different from other regular AI integrations like assistants mostly in terms of higher degree of autonomy and complexity. Basically, LLMs can generate instructions or actions in text format, but they cannot perform these actions directly. A mechanism is needed to execute these actions and provide results back to the LLMs. The LLM then decides (based on rules/learning/etc.) whether the result achieves the goal, or if further action is required. When the goal is achieved, the loop ends. E.g. a regular support chatbot can respond to user queries based on configured tools (vector databases), and generate a response. An AI agent could proactively monitor system logs, identify potential security threats, and take corrective measures without human intervention.
Consider the following pseudocode for an agent that analyzes audit logs and creates tickets and blocks access.
const openai = require('openai')
async function detectAnomaly(logFileContent) {
const prompt = `Analyze the audit entries in this log file and when you potential anomalies, respond back in JSON format with user field with username and anomaly field set to true: ${logFileContent}`
const response = await openai.createCompletion({
model: 'text-davinci-003',
prompt: prompt,
temperature: 0.5,
max_tokens: 60,
})
return response.data.choices[0].text.trim()
}
//read log file
const logContent = getLogContent() //read/stream form wherever, chunk, etc.
detectAnomaly(logEntry)
.then((result) => {
const response = JSON.parse(result)
if (response.anomaly === true) {
console.log('Anomaly detected for user', response.user)
// create ticket
// block user
// etc.
} else {
console.log('No anomaly detected.')
}
})
.catch((error) => {
console.error('Error:', error)
})
AI use cases are evolving and innovation is ongoing, but on a high level, we can categorize agents as following.
1. Rule-Based Agents These agents operate on predefined rules and logic. They excel in scenarios with clear, unchanging rules and limited complexity. Simple chatbots that run based on conditional logic like if a then b, etc. are examples of rule based agents. Another example could be used by IT teams that renew subscriptions, reorder supplies, etc. based on some rules.
2. Reactive Agents These agents respond directly to triggers without maintaining any internal state. The behavior is purely determined by the current input (Stateless). Self driving cars need obstacle prevention systems to avoid collisions and can use these agents. In the data protection domain, based on ransomware detection, a reactive agent could put in measures to prevent the spread by isolating the network for example.
3. Goal-Based Agents Goal-based agents possess internal goals and plan their actions to achieve those goals. Current state and potential next state or states determine the next action, out of different actions available. Think of chess. Or courier delivery service like UPS that have to decide the route based on optimal route, whether, road blocks, etc.
4. Learning Agents Learning agents adapt and improve their behavior through experience and interaction with the environment. It's basically machine learning technique to learn from data and optimize decision-making. Example could be recommendation systems that read user behavior (likes, past history, etc.) and present recommendations. In the data protection domain (which is my favorite :D), a ransomware detection agent could analyze network traffic and data to detect something's off.
5. Hybrid and other Agents I believe with innovation and new use cases coming forward, we will see more categories. Hybrid agents would combine aspects of the above agent types to leverage individual strengths and address more complex scenarios. Smart home systems is a good example that can use rule-based automation (turn off lights after 10) and learning (cooling temperature based on preference).
Beyond these traditional classifications, modern AI agents leverage frameworks like ReAct (Reasoning and Acting). This approach interleaves thought (reasoning using Large Language Models) and action (executing tasks via external tools), enabling agents to dynamically adapt and make informed decisions in complex scenarios.
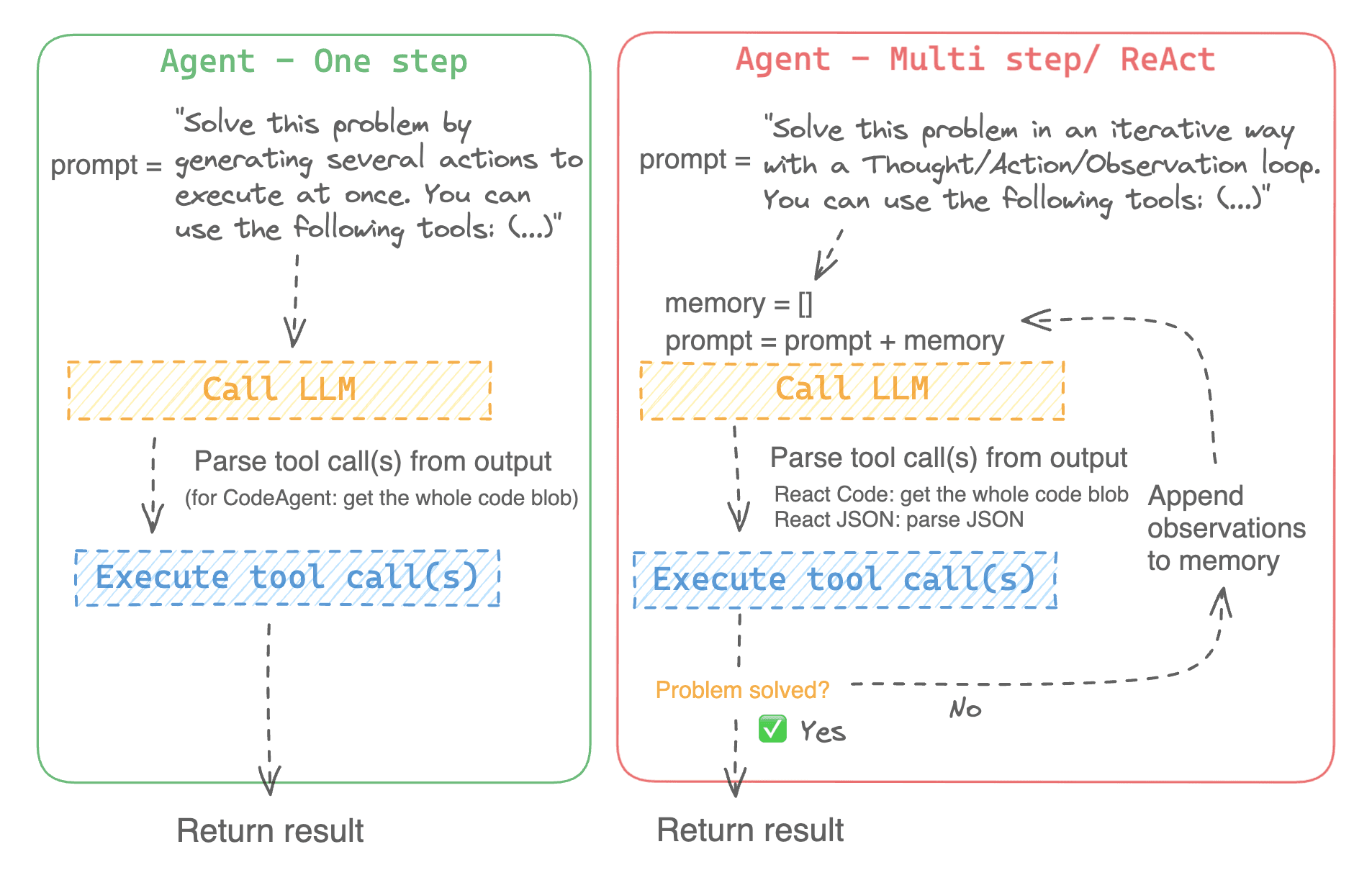
This classification is more from the underlying mechanisms and capabilities perspective. From application and domain angle, Thomas Kurian, Google Cloud CEO talks about the following agent types.
1. Customer Agents Working across channels and integrated into your product experiences, these agents that can listen, understand needs, and make recommendations.
2. Employee Agents These agents help workers be more productive and collaborate better by streamlining repetitive tasks, answering questions, and editing communications.
3. Creative Agents These agents support creative design and tasks that need creative inputs (marketing, etc.).
4. Data Agents Data agents act on your own data, research it and can answer questions, synthesize research, and provide insights.
5. Code Agents These agents can generate code, do code reviews and other tasks to support developers.
6. Security Agents These agents assist automate monitoring and data protection tasks.
This article, show cases close to 100 real world use cases of various agents types.
Tools/Frameworks
In the near future, as we see more adoption and use cases, there will be more high level frameworks that will allow developers to write these agents and cloud support to deploy them. Here're some open source and commercial frameworks to check out.
- AutoGPT
- AgentGPT
- Semantic Kernel
- LlamaIndex Agents
- Microsoft AutoGen framework
- Google's Vertext AI Agent Builder
- Agent-OS
- LangChain Agents
Let me know if you have built any AI agents? What tools/frameworks did you use?